Introduction
This article shows how to configure HttpClient to send a certificate to an API that uses certificate authentication.
Getting the Certificate
The Owner of the API should provide the certificate in pfx format which is a file that contains the certificate along with the key for the certificate this file can be protected by a password that can be set during the creation of the pfx file.
What is PFX file?
A PFX file, also known as PKCS #12 , is a single, password protected certificate archive that contains the entire certificate chain plus the matching private key. Essentially it is everything that any server will need to import a certificate and private key from a single file
Why Do I need a PFX file?
As we will see in the next part, we are going to use X509Certificate2 which will be used to open the certificate file and decrypts the private key and saves it to a key container.
I have CRT and KEY file can I generate a PFX file
Yes, you can do that, using openSSL Command we can combine the CRT file and the KEY files in a single PFX file protected by a password.
Here is the command that I have used to generate the PFX file:
openssl pkcs12 -export -in [CRT_FILE] -inkey [KEY_FILE] -out [OUT_PUT_FILE_NAME]
example:
openssl pkcs12 -export -in ./Certificate.crt -inkey ./Certificate.key -out ./Certificate.pfx
after executing the command, you are prompt to enter the password and verify the password then the PFX file is generated.

Using the Certificate in C#
Below are a sample code that uses the generated certificate in HttpClient:
private async Task<JsonDocument> GetApiDataUsingHttpClientHandler()
{
var cert = new X509Certificate2(Path.Combine(_environment.ContentRootPath, " Certificate.pfx"), "123456");
var handler = new HttpClientHandler();
handler.ClientCertificates.Add(cert);
var client = new HttpClient(handler);
var request = new HttpRequestMessage()
{
RequestUri = new Uri("https://localhost:44379/api/values"),
Method = HttpMethod.Get,
};
var response = await client.SendAsync(request);
if (response.IsSuccessStatusCode)
{
var responseContent = await response.Content.ReadAsStringAsync();
var data = JsonDocument.Parse(responseContent);
return data;
}
throw new ApplicationException($"Status code: {response.StatusCode}, Error: {response.ReasonPhrase}");
}
Source Code
Full source code for the above sample can be found here on git hub.
Add new comment

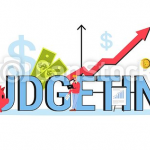
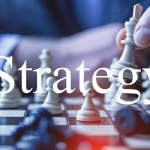